AWS Cognito is one of the useful Amazon cloud services available for developers. This service allows you to connect it with other available services on AWS such as Lambdas, AppSync, or API Gateway in a few steps. AWS Cognito stores personal user data — full name, age, email, username, location, password, and all user form data which your web or mobile application collects. In this article, we will share what AWS Cognito is, how to use AWS Cognito and Amazon Cognito implementation examples.
Amazon (AWS) Cognito Implementation Best Practices
What is AWS Cognito
AWS Cognito was created for easier user registration and logging into your application or webpage, their personal information storage, and some of the features like two-factor authentication or password recovery procedure. This service is quite a good solution for these purposes. Thanks to AWS Cognito features, your development team avoids writing a backend service. Moreover, this service allows you to replace a large amount of frontend code with a more readable amount of code. On the other hand, you need to clearly understand in what situations and why this service can be used, so we recommend that you thoroughly study the pros and cons of using it before making a decision.
It is a fully managed identity service from AWS. AWS Cognito for web applications provides easy and secure user registration, login, access control, token renewal, and user identity management. Cognito scales to millions of users and supports logins with social media providers like Facebook, Google, and Amazon. You can also use your own or external identity provider that supports SAML or OpenID Connect standards.
1. The client requests a token;
2. The Cognito User Pool verifies the user against the identity provider or does it on its own;
3. Cognito User Pool returns a token to the client;
4. The client uses the token to access the application.
Cognito has two main parts: user pools and identity pools.
User Pools
User pools provide a secure user directory that stores all of your users and scales to a significant number of users. As a serverless technology, custom pools are easy to configure without the worry of maintaining any infrastructure. User pools are what govern all users who register and log into an account, and are the main part we will focus on in this article.
Identity pools
Identity pools allow you to authorize users logged into your application to access various other AWS services. Let's say you want to give the user access to a lambda function so they can get data from another API. You can specify this when creating an identity pool. User pools include the fact that the source of these identifiers could be a Cognito user pool or even a Facebook or Google user pool.
Cognito User Pools allows your application to call various methods on a service to manage all aspects of user authentication, including things like:
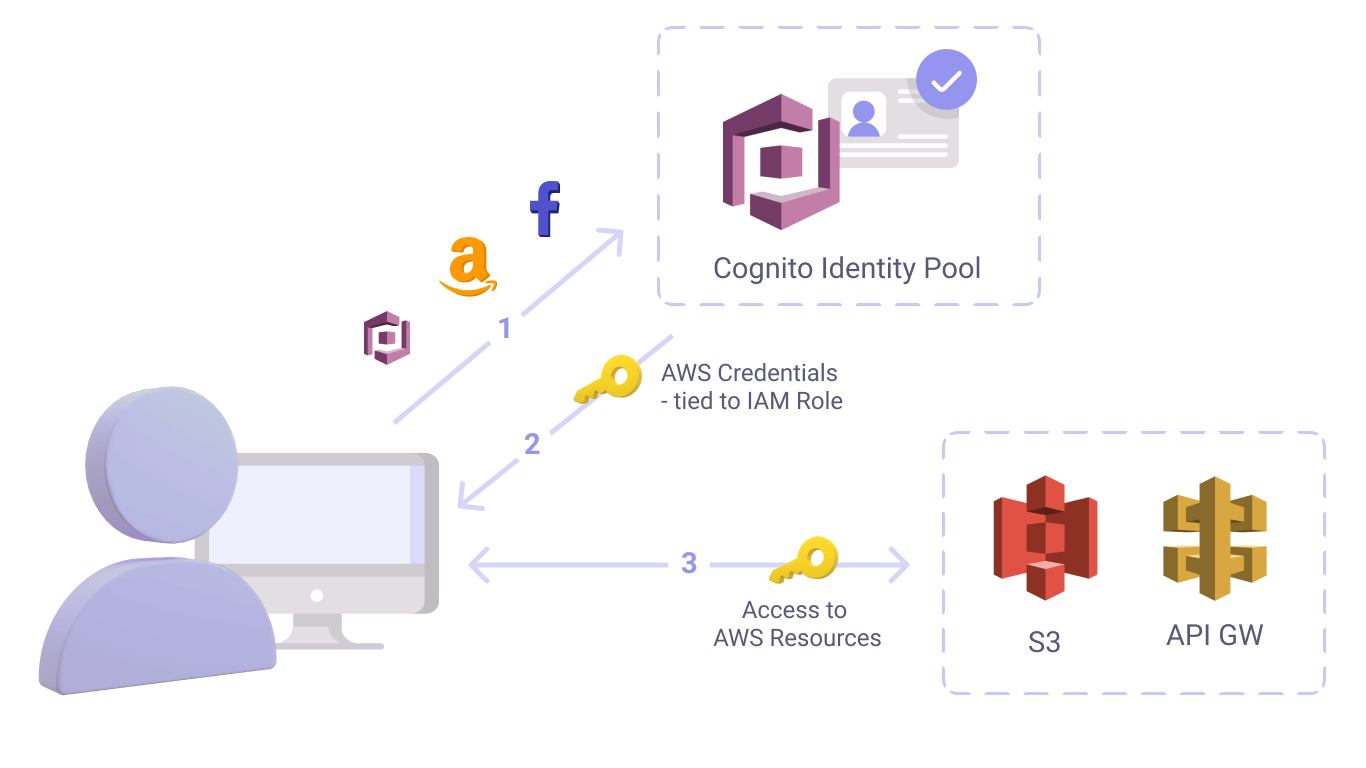
1. User registration
2. User login
3. User logout
4. Change user password
5. Resetting user password
6. MFA Code Verification
7. AWS Cognito Integration with AWS Amplify
AWS Amplify supports AWS Cognito for mobile apps in a variety of ways. First of all, you can create and configure Amazon Cognito services directly from the AWS Amplify CLI. Once you create an authentication service through the CLI, you can call various methods (for example, signUp, signIn, and signOut) from your JavaScript application using the Amplify JavaScript client library.
Amplify also has pre-configured UI components that allow you to build entire authentication flows in just a couple of lines of code for frameworks like React, React Native, Vue, and Angular.
How authorization works
Upon authorization, the user is given three tokens:
1. Id_token - token with user information
2. Access_token - token for user identification
3. Refresh_token - token for updating id_token and access_token
Id_token and access_token are issued for one hour. Refresh_token is issued for a month. You can find more details about how authorization works here.
AWS Cognito Benefits
1. All settings can be made through a single AWS control panel
2. Easy connection to most popular frameworks and libraries like Vue, Angular, React, Nodejs via provided AWS Amplify module
3. Having its own global state that can be used for the entire project, which eliminates the need for an additional solution for managing the global states of the application
4. Ready-made templates for registration forms, login, password recovery, password change, MFA (Multi-Factor Authentication) e.g. SMS, Email, and TOTP (Temporary One Time Password) Confirm MFA Code’s and Provide QR codes for TOTP
5. All user information is stored in the cloud AWS Cognito service, which allows you to quickly get the data you need.
6. Automatic email to confirm user registration and automatic text message to verify mobile number.
7. The ability to store custom user attributes such as name, age, phone number, address, etc.
8. Secure and encrypt data sent from the application by AWS Amplify module
9. Easy AWS Cognito integration with different AWS services like AWS AppSync
10. Managing UI Form validations with AWS control console
11. Integration with Social login providers e.g. Google or Facebook accounts.
12. Ready-made error messages
13. Several different possibilities to handle forms on the application side
14. An opportunity to make HTTP requests with the help of AWS Amplify (it’s using Axios module under the hood)
15. Industry-standard security features like throttling (to prevent brute force attacks) or tokens refreshing (to allow revoking access tokens)
The cons of AWS Cognito
1. This solution requires financial investment, after 50k MAU (monthly active users) you should pay for each user.
2. Not very detailed documentation, which is rarely updated.
3. Some options can be set only during the creation of an AWS Cognito user pool in the AWS administration panel. After that those options are disabled and they can't be changed easily. If you need to make changes, you should delete the whole instance and create a new one. This can be a little uncomfortable at the very beginning of the project — when you are not sure which options you will eventually use in production.
4. Aligning provided UI forms with some of the designs can sometimes be problematic, then probably the fastest way is to create your own form components
5. Error messages provided by AWS Cognito are not very user-friendly. Sometimes they are too technical, so you need to provide some kind of an error mapper in the application, to show more user-friendly messages. For example when a user tries to log in with the wrong password.
6. There are no error messages for specific form fields, only general error messages
7. Confirmation emails (after user registration) are very limited. You need to create custom HTML email templates if you want more than just a plain text email with a verification link
8. There are limitations in the number of custom field attributes. You can’t create more than 25 custom attributes.
9. AWS Amplify module is a little heavy (minified + gzipped version is around 180 kB)
Lambda function for user registration and authentication
One of the AWS Cognito best practices is AWS serverless Cognito integration with Lambda functions. With the help of the Lambda function, you can do the following actions for calling the Amazon Cognito API:
1. Registration (email/SMS message with a confirmation code) / users / register
2. Confirmation of registration (from the code sent to the mail) / users / confirmRegistration
3. Resend code (email or SMS) / users / resendCode
4. Authentication (by login and password, but federated access can also be used) / users / authenticate
5. ForgotPassword Reminders / users / forgotPassword
6. Confirm new password / users / confirmNewPassword
7. Logout / users / logout
An example of a user registration method:
public StringMap register(String username, String email, String password) throws Exception {
List userAttributes = new ArrayList<>();
userAttributes.add(new AttributeType().withName(awsProperties.getAttributeEmail()).withValue(email));
SignUpRequest signUpRequest = new SignUpRequest()`
.withClientId(awsProperties.getAppClientId())
.withSecretHash(getSecretHash(username))
.withUsername(username)
.withPassword(password)
.withUserAttributes(userAttributes);
try {
awsCognitoIdentityProvider.signUp(signUpRequest);
} catch (InvalidParameterException e) {
return createStatusFail("register.FAIL", e.getErrorMessage());
} catch (UsernameExistsException e) {
return createStatusFail("register.FAIL", e.getErrorMessage());
}
return createStatusOk("register.CONFIRM_EMAIL", "Registration succeed. Confirmation code was sent on your email.");
}
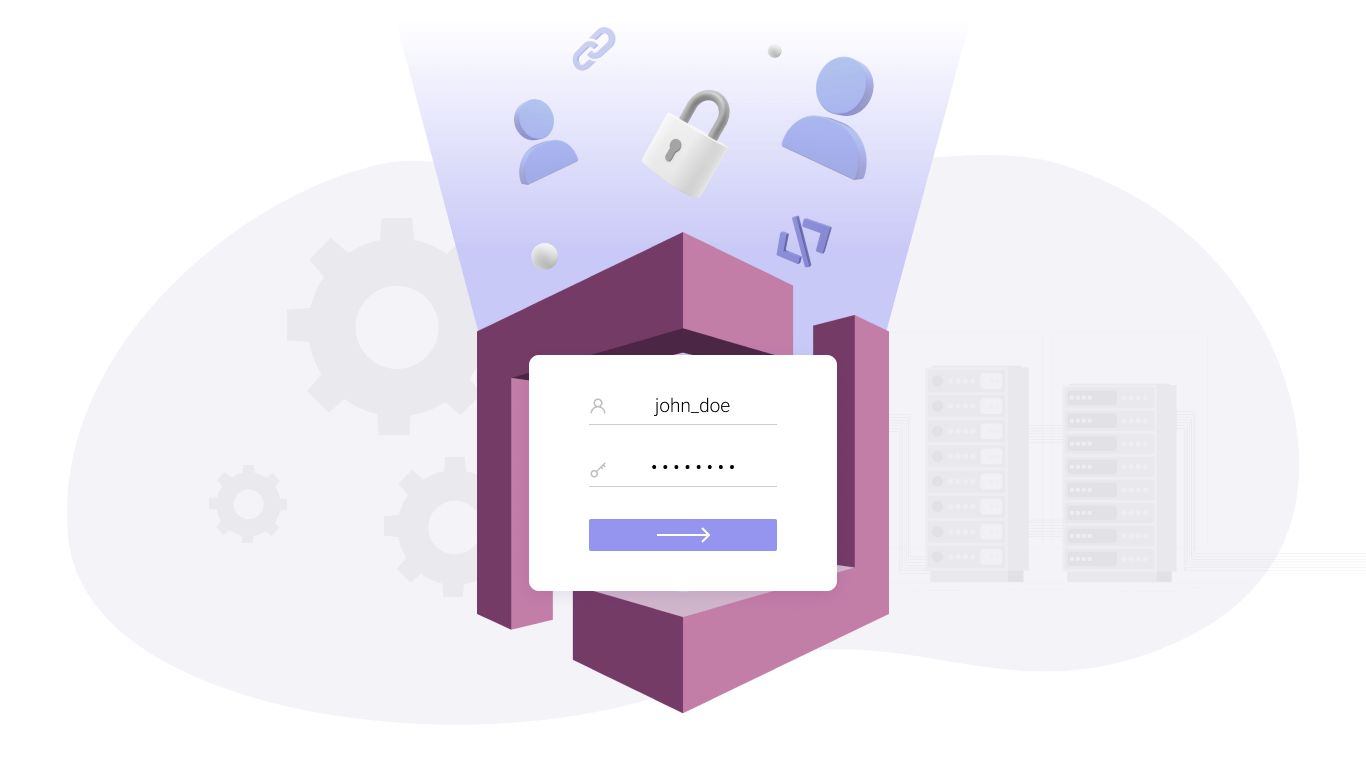
Cognito authentication strategies
One of the powerful tools in AWS Cognito is triggering AWS Lambda functions during user pool operations such as user sign-up, confirmation, and sign-in (authentication) with a Lambda trigger. It's not only logic that Lambda triggers allow to add to sign in or sign up flows, they also create new approaches to user authentication. One such approach is passwordless authentication. This approach is aimed at getting rid of passwords and replacing them with a one-time password sent to the user’s phone number or email.
Thus the client does not need to update and remember a new password every few months, and the possibility of a stolen password is reduced to zero.
Another approach is authentication and authorization of users through social profiles such as Facebook, Google, Login with Amazon and Log in with Apple. This approach simplifies the process of user registration and logging into the account by obtaining information directly from the social profiles with security best practices.
Geniusee has extensive expertise in building both traditional and non-standard authentication approaches using AWS Cognito, including social profile authentication and passwordless authentication using native AWS services such as AWS SNS for SMS or AWS SES for emails and third party services such as Twillio, Sendgrid, Mailgun, etc.
AWS Cognito implementation
To integrate the AWS Cognito service into your application, you just need to import the AWS Amplify module which is available as the NPM module (Node Package Manager). It gives your software project the ability to connect with the AWS Cognito service, and implement the native user interface forms for authentication, password recovery, etc.
Pricing
When you use Amazon Cognito Identity to create your user pool, you only pay for your monthly active users (MAU). MAUs are users who have performed at least one identification operation during a calendar month: registration, authorization, token renewal or password change. Subsequent sessions of active users and inactive users in this calendar month are not charged.
Our Experience
Geniusee is proud to have a strategic partnership with Amazon Web Services (AWS). The partnership will help Geniusee and AWS accelerate customer innovation and make the best use of the cost-effectiveness and flexibility of the Cloud.
Enterprise customers increasingly rely on AWS expertise to achieve targeted business results faster by migrating to a modern, optimized cloud platform. Geniusee has broad experience in a migration strategy, cloud implementation, and cloud infrastructure cost optimization. This interaction will provide access to the best technical resources, which will allow customers to have a clear understanding of the installed software, the existing IT infrastructure, and its effective management in the Cloud.
Research and consulting firm Gartner Group, Inc. predicts that cloud infrastructure spending will grow by nearly 60% over the next two years, while investment in data center systems will decline. This means organizations will be careful about their IT investments. Geniusee can provide guidance, tools, and expertise to manage costs and security in the Cloud.
Over the past few years, Geniusee has been developing and diversifying its cloud services. Both Geniusee and AWS expect the collaboration to bring added value to customers who are looking to fully optimize their IT infrastructure using best practices in cloud adoption.
Final thoughts
Using AWS Cognito for user authentication along with AWS Amplify on the application side is probably a better and faster solution than creating the whole user and server side authentication service from scratch. In addition, you don’t need to take care of the backend, because this will be already provided by AWS Cognito. Also, some of the error messages, connection with service, UI Cognito forms will be provided out of the box. This is a good choice if you want to use advanced security features like phone number verification, two-factor authentication, or log in with Google/Facebook.
But if your application does not use any other service from AWS (besides Cognito), connecting it with third-party services will take some time and require custom solutions. In this situation, it’s definitely better to consider building a dedicated authentication service or use an alternative solution that will fit with the rest of the application services/features.
Воок a qoute with our experts if you need help from AWS certified specialists info@geniusee.com.