We continue our Interview Questions rubric. Today we’ll talk about Java interview questions: both basic and advanced questions and answers for developers.
Top 20 Java Developer Interview Questions and Answers: Everything You Should Know Before Your Job Interview
Introduction
Preparation for the interview might be stressful and discouraging, especially if you lack some information or experience. That’s why this tutorial might benefit you a lot, at least giving you the core directions to recall from your personal database. Moreover, here you will find not only the questions, but the short answers with the code examples included. So, if you are already an old Java soldier, this article will just refresh everything you already know, and if you are a brave rookie, it will put your knowledge together and help you be on fire during your interview.
In this article, we will talk about Java 8 version. First, some basic java developer interview questions will be covered, and then we will switch to more advanced ones.
Let's start from the beginning and move on to the basics.
Question 1: What is Java?
This seems like one of pretty general java interview questions, right? Well, then you should get a clear and unambiguous answer to it. Here is one of the options.
Java is an object-oriented, secure, and high-level programming language. It was created and developed back in 1991 by a man named James Gosling. The Java language is developed under the slogan “WORA” - “write once, run anywhere”. It is known for its flexibility and high efficiency.
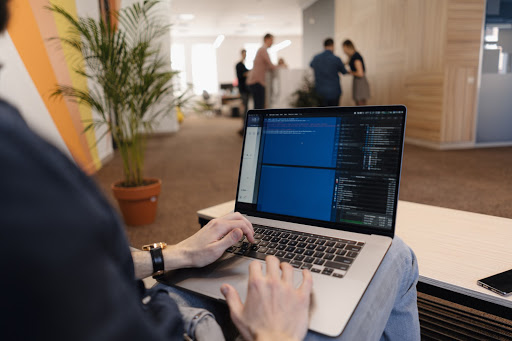
Question 2: Is Java a fully object-oriented language?
This is a good Java interview question, and no, it is not fully object-oriented. Java uses some data types (char, byte, float) that are not objects.
Question 3: What are some of the benefits of Java?
With this question, the interviewer is trying to define the depth of your experience in Java, your analytical skills, and your general competency. You can stress the most significant advantages compared with the other programming languages basing on your experience. It will be greatly appreciated if you provide several examples.
For novice programmers and developers, the most important feature is simplicity. It is believed that Java functions are fairly easy to learn, especially when compared to other programming languages.
The Java programming language is also considered very safe compared to other programming languages. This is achieved through an interpreter called the JVM — this interpreter is installed with Java itself, and it constantly provides your computer with the latest security updates from the Internet.
In addition, Java is fully adaptable. Thanks to the “WORA” principle, on which the Java language is based, it can be transferred and used on any computer and operating system that you need - it is completely flexible and multifunctional.
Question 4: What is the feature of Java 8?
In Java, the developer must clearly answer the comparison questions like this. This is one of the largest language updates, so it would be nice to know what they are.
Java 8 introduced language support for String, provided an improved Date / Time API, and contributed to the development of the JVM, a system responsible for many things in Java, including Java's security functions. In general, Java 8 has made the programming language more accessible and more similar to modern programming languages.
Question 5: What are access specifiers in Java?
There are four access specifiers which the candidate should understand. There are the following qualifiers: public, private, protected and standard.
Public qualifiers allow access to any class or through any method (hence their name). In contrast, private specifiers only allow access within the specified class itself. Protected ones open access that somehow correlates with the class - either from within the class, from a subclass, or simply from the same package. Finally, default denotes a standard area and allows access from only one package.
Question 6: What is a constructor?
A constructor is a piece of code that initializes a specific object. The Java programming language has two types of constructors - default and modified.
Question 7: What is an Object?
A general question in a Java job interview. An object in Java has a state and behavior. The most common and understandable definition of an object is that it is a type of class.
Question 8: What object references can be in Java?
This is one of the simplest questions - in Java, all object references are null.
Question 9: What is the difference between JDK, JVM, AND JRE?
This type of technical question gives you the wide space to demonstrate your strong knowledge of the subject. The best way to answer the questions like this is to define each of the terms, then move to their functions and finish up with the biggest differences. Also, you may do well if you describe the way these terms are related.
JDK (Java Development Kit) is a Java development kit. This is the main Java function tool used to compile programs in this language. The package has all the necessary tools to start using it.
We have already mentioned JVM (Java Virtual Machine) earlier - this means Java Virtual Machine. This is a machine that creates an environment in which Java bytecode is handled properly.
JRE (Java Runtime Environment) is a Java runtime environment. This is the type of environment provided by the JVM — it allows Java bytecode to work properly.
Question 10: Custom exception in Java: why and how to create it?
This operational question provides the interviewer with extra information about how you go in performing the tasks, which are required by the position you’re going to occupy. Answer this question directly and briefly.
You create custom exceptions in order to increase the flexibility and add extra methods, attributes. In general, it is a way to meet the specific user’s needs. You can make it possible by expanding the java.lang.Exception class.
Now that we have covered some of the basic issues, we can move on to questions for experienced developers and programmers. These questions will mainly be related to code.
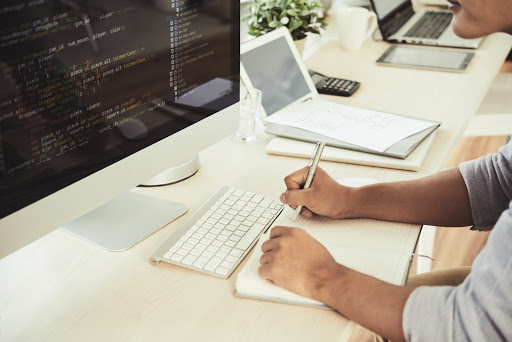
Advanced questions
Question 1: What is the differenct between ‘method overloading’ and ‘method overriding’?
This can be one of the first interview questions for java developers.
In the case of ‘method overloading’, methods belonging to the same class have the same name, but their parameters are different. This applies to extensions of the behavior of the method more than anything else. Conversely, ‘method overriding’ subclasses have methods with the same names and parameters. The goal here is to change the behavior of an existing method.
Question 2: What is the conclusion of this Java program?
public class Test
{
Test (int x, int y)
{
System.out.println ("x =" + x + "y =" + y);
}
Test (int x, float y)
{
System.out.println ("x =" + x + "y =" + y);
}
public static void main (String args [])
{
byte x = 30;
byte y = 65;
Test test = new Test (x, y);
}
}
The correct answer looks like this:
a = 30 b = 65
Question 3: Is it possible to run the program without the ‘main () method’?
A pretty standard Java interview question and yes, it is possible. One of the most common ways to run such a program is to use a static block.
Question 4: What is ‘runtime polymorphism’?
Runtime polymorphism is a process in which a particular call sent using a specific method will be resolved at runtime, rather than at compile time. Here is an example:
class Tree {
void run ()
{
System.out.println (“tree is standing");
}
}
class Willow extends Tree {
void run ()
{
System.out.prinltn (“willow is standing on a hill");
}
public static void main (String args [])
{
Tree b = new Willow (); // upcasting
b.run ();
}
}
Question 5: What is ‘Inheritance’?
This term, frankly, lies on the surface. Inheritance is when one object acquires the properties and parameters of another (another class). The override method discussed above uses this. The basic idea of inheritance is that you can create new classes on existing ones. There are five different types of inheritance, but the Java programming language only supports four (multiple inheritance is not supported). Why is multiple inheritance not supported? In fact, there is only one specific reason - to simplify the program. This should be an important note that the candidate is advised to remember.
Question 6: What is the largest class (SuperClass) that exists in Java?
This is one of the easiest questions. The largest in Java is the object class.
Question 7: What does the Super prefix mean in Java?
Super in Java is used as a reference to point to the immediate parent of the class. You can also use the command to invoke the immediate parent methods of the class and constructor.
Question 8: What will be the result of this program?
class Animal
{
public Animal ()
{
System.out.println ("Animal class constructor called");
}
}
public class Zebra extends Animal
{
public Zebra ()
{
System.out.println ("Zebra class constructor called");
}
public static void main (String args [])
{
Zebra e = new Zebra ();
}
}
Here is the answer:
Animal class constructor called
Zebra class constructor called
Question 9: What is ‘association’ in Java?
This is one of the frequently asked Java engineer interview questions. At first glance, it may seem that it is quite simple. However, things like aggregation and composition come from the association, so it’s important that the developer clearly understands this term.
An association is when all objects have their own life cycles, and a specific owner does not exist. It can range from “one” to “many”.
Question 10: What is ‘object cloning’?
The ‘object cloning’ command is used to create an identical copy of an object. This is done using the clone () method from the object class.
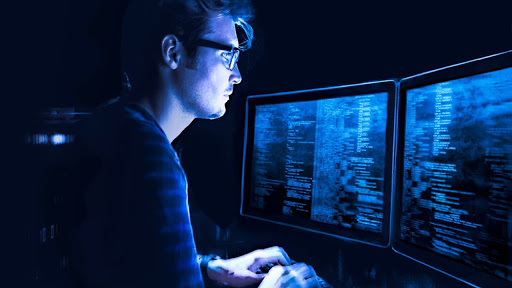
Conclusion
Programming specialists are always in demand. Java is one of the most popular specific programming languages in the world (due to its flexibility, security and simplicity). In this article, we have looked at the java developer interview questions and answers that will help you evaluate a candidate’s knowledge.
All that remains for you to do is to hold an interview and select a competent employee for the position of Java developer and strengthen your team of experienced professionals! And the final advice:
Consider Geniusee Your Trusted Partner
If you look forward to taking next career steps, consider teams that grow talents and value people. We at Geniusee are the great example. A team of dedicated professionals with a big practical experience in full-cycle software development. Our experts are always ready to analyze your idea and select the right technology for the particular project and cooperate in partnership and trust.
You can get inspired by our work results here and consider us either your ground for personal and professional development or your reliable partner in business.