The Swift programming language is only five years old, but it is already becoming the main development language for iOS. Developing to version 5.2, Swift has become a complex and powerful language that meets both object-oriented and functional paradigm. And with each new release, it adds even more features.
16 Most Asked Questions for iOS Developer in Interview
But how well does the candidate really know Swift? In this article, you will find sample questions for a Swift interview.
Questions are divided into two groups:
- Beginner: suitable for those who are really interested in the language. The candidate has already read several books about this language and has already begun experimenting with it.
- Advanced: suitable for the most advanced developers, who like to get into the jungle of syntax and use advanced techniques.
Questions for beginners.
Question 1: What is optional and what problems does it solve?
Optional allows a variable of any type to present a "no value" situation. In Objective-C, "no value" was only available in reference types using the special nil value. Value types, such as int or float, did not have this capability.
Swift has extended the concept of "no value" to value types. The optional variable can contain either a value or nil, indicating the absence of a value.
Question 2: Enumerate briefly the main differences between structure and class.
Classes support inheritance, but structures do not.
Classes are a reference type, structures are a value type.
Question 3: What are generics and what are they for?
In Swift, you can use generics in classes, structures, and enumerations.
Generics fix code duplication. If you have a method that accepts parameters of one type, sometimes you have to duplicate code to work with parameters of another type.
For example, in this code, the second function is a “clone” of the first, except that it has string parameters, not integers.
func areIntEqual (_ x: Int, _ y: Int) -> Bool {
return x == y
}
func areStringsEqual (_ x: String, _ y: String) -> Bool {
return x == y
}
areStringsEqual ("ray", "ray") // true
areIntEqual (1, 1) // true
Using generics, you combine two functions in one and at the same time ensure type safety:
func areTheyEqual < T: Equatable> (_ x: T, _ y: T) -> Boo
return x == y
}
areStringsEqual ("ray", "ray")
areIntEqual (1, 1)
Since you are testing equality, you are restricting types to those that conform to the Equatable protocol. This code provides the desired result and prevents the transfer of parameters of the wrong type.
Question 4: In some cases, you cannot avoid implicitly unwrapped optionals. When and why?
The most common reasons for using implicitly unwrapped optionals:
- when you cannot initialize a property that is not nil at the time of creation. A typical example is the outlet at Interface Builder, which is always initialized after its owner. In this special case, if everything is configured correctly in Interface Builder, you are guaranteed that the outlet is non-nil before using it.
- to solve the problem of the strong reference loop when two instances of classes reference each other and a non-nil reference to another instance is required. In this case, you mark the link on one side as unowned, and on the other side use implicit optional expansion.
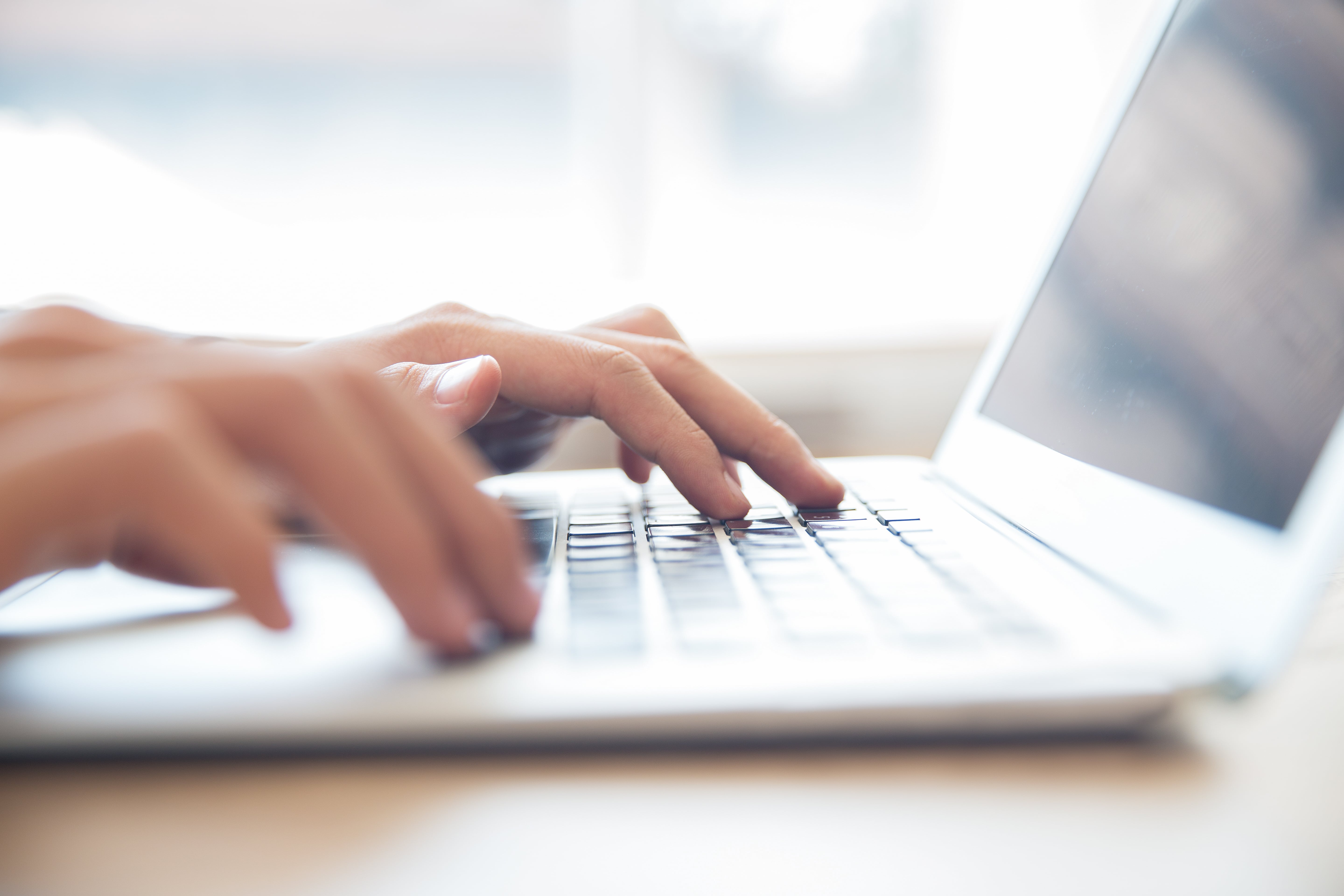
Question 5: How can we deploy optional? Rate them in terms of security
Fvar x: String? = "Test"
The most common reasons for using implicitly unwrapped optionals:
- Forced unwrapping is insecure. let a: String = x!
- Implicit deployment when declaring a variable is unsafe. var a = x!
- Optional binding is safe. if let a = x {
print ("x was successfully unwrapped and is = \ (a)")
} - Optional chaining is safe. let a = x? .count
- Nil coalescing operator is safe. let a = x ?? ""
- The Guard statement is safe. guard let a = x else {
return
} - Optional pattern - safe.if case let a? = x {
print (a)
}
Question 6: Is it possible to add a stored property to a type using extension? How or why not?
No, it is impossible. We can use extension to add new behavior to an existing type, but we cannot change the type itself or its interface. To store the new stored property, we need additional memory, and extension cannot do this.
Question 7: What is a protocol in Swift?
A protocol is a type that defines an outline of methods, properties, etc. A class, structure, or enumeration can take a protocol to implement all of this. The protocol itself does not implement the functionality, but defines it.
Question 8: Consider the following code:
struct Tutorial {
var difficulty: Int = 1
}
var tutorial1 = Tutorial ()
var tutorial2 = tutorial1
tutorial2.difficulty = 2
What are the values of tutorial1.difficulty and tutorial2.difficulty? Would there be any difference if the Tutorial was a class? Why?
tutorial1.difficulty is 1, and tutorial2.difficulty is 2.
In Swift, structures are value types. They are copied, not referenced. The following line copies tutorial1 and assigns it to tutorial2:
var tutorial2 = tutorial1
Changes in tutorial2 do not affect tutorial1.
If Tutorial were a class, tutorial1.difficulty and tutorial2.difficulty would equal 2. Classes in Swift are reference types. When you change the tutorial1 property, you will see the same change for tutorial2 - and vice versa.
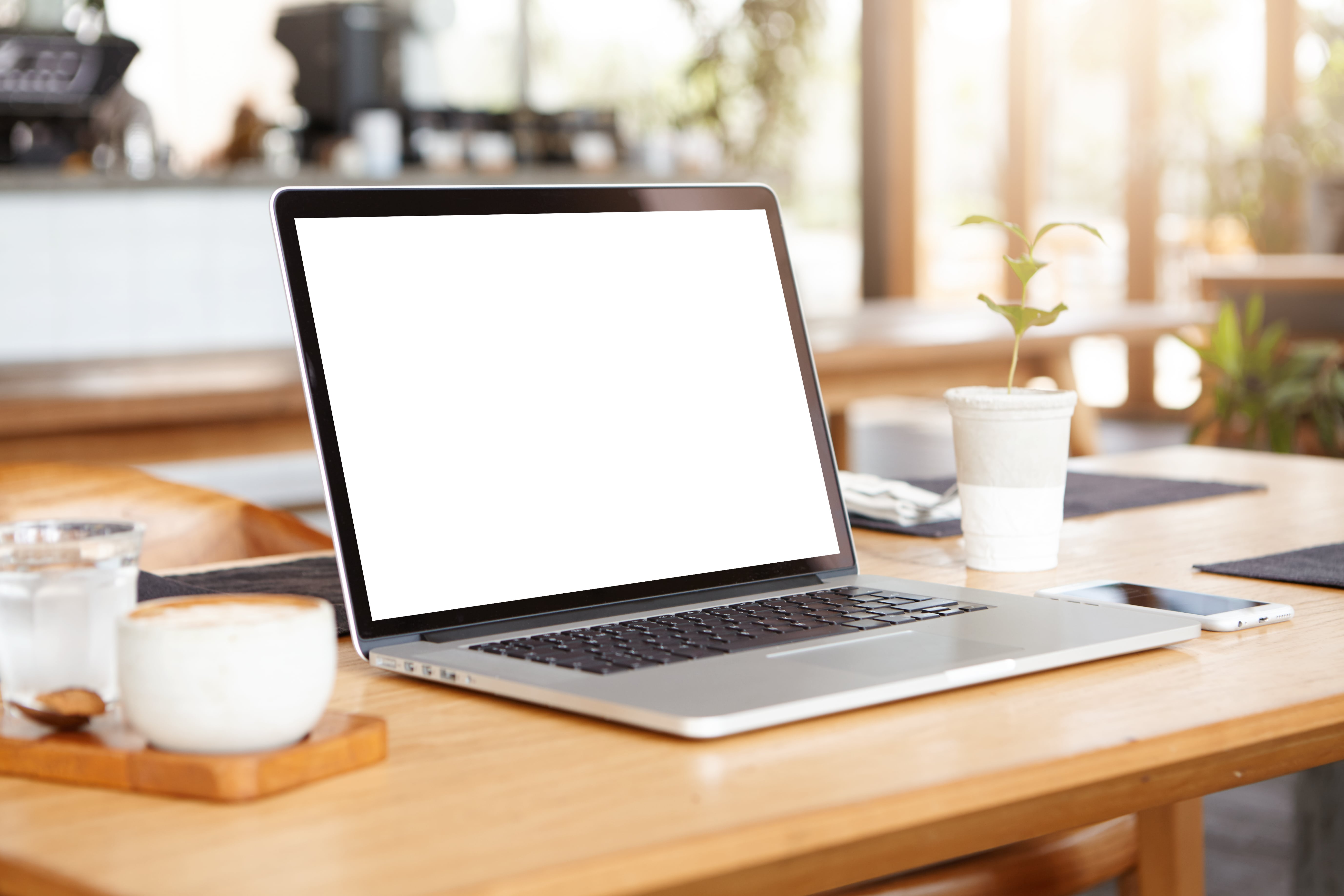
Advanced questions.
The iOS interview doesn't end there. Questions can be more complicated. Let’s move to the advanced level.
Question 1: Is Swift an OOP language or a functional programming language?
Swift is a hybrid language that supports both types. It implements the three basic principles of OOP: Encapsulation, Inheritance, Polymorphism.
As for Swift as a functional language, there are different, but equivalent ways of defining it.
One of the most common on Wikipedia runs as follows: "... a programming paradigm that considers computing as an estimate of mathematical functions, avoids changing states and mutable data."
It cannot be argued that Swift is a complete functional language, but the basics are present.
Question 2: In Objective-C, a constant can be declared as follows: const int number = 0;
This is an option for Swift: \let number = 0
Is there any difference between the two? If so, can you explain how they differ?
Sometimes an iOS interview includes tricky questions like this.
A constant is a variable initialized to a compile-time value or an expression allowed at compile time.
A constant created through let does not have to take a value at compile time, but there is still a condition: a value can only be determined once.
Question 3: Can you add a saved property using the extension? Explain why / why not.
No, it is impossible. An extension can be used to add new behavior to an existing type, but not to change the type itself or its interface. If you add a saved property, you will need additional memory to store the new value. The extension is not designed for such tasks.
Question 4: Are trailing expressions elements (values) or reference types?
Trailing expressions are reference types.
Question 5: Describe the situation when you can get a circular reference in Swift?
A loop reference is possible when two instances contain strong references to each other, causing a memory leak, since neither of the two instances will ever be freed. The reason is that the instance cannot be freed as long as there is a strong reference to it.
You can solve the problem by replacing one of the strong links with weak or unowned.
Question 6: Swift allows you to create recursive enumerations.
Here is an example of such an enumeration that contains a Node variant with two associative types, T and
List: enum List <T> {
case node (T, List <T>)
} There will be a compilation error. What did we miss?
We forgot the indirect keyword, which allows similar recursive enumeration options:
enum List <T> {
indirect case node (T, List)
}
Question 7: Explain Core Data.
Core data is one of the most powerful frameworks provided by Apple for macOS and iOS apps. Core data is used for handling the model layer object in our applications. We can treat Core Data as a framework to filter, modify, save, track the data within the iOS apps. Core Data is not a relational database.
Using core data, we can easily map the objects in our app to the table records in the database without knowing any SQL. Core data is the M in MVC structure.
Question 8: What are the different ways to pass data in swift?
There are several ways to pass data in swift such as KVO, Delegate, NSNotification & Callbacks, Target-Action, etc.
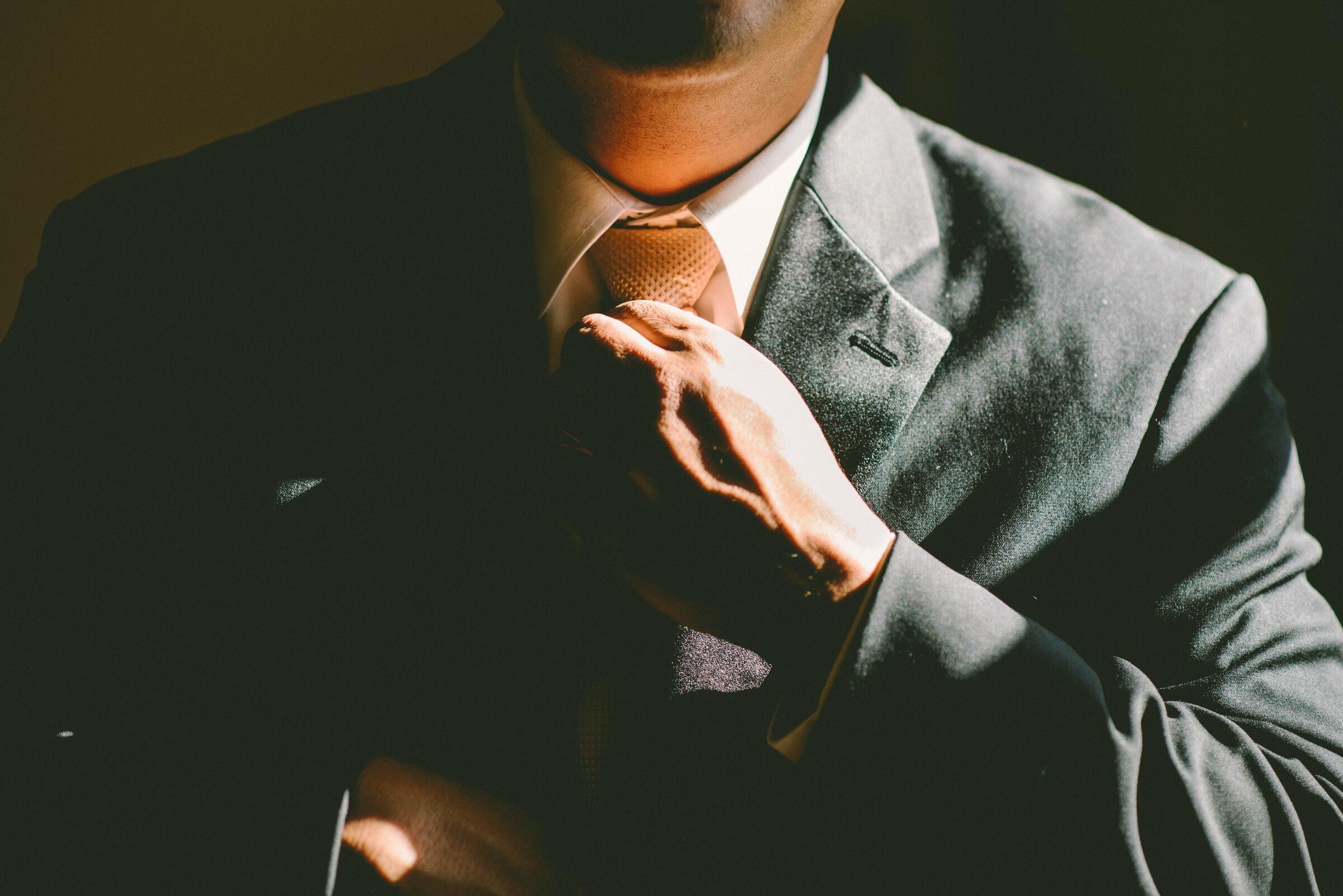
Conclusion
We hope that the answers provided here would indeed be helpful in understanding the iOS swift basics and advanced topics. These Swift developer interview questions would assist you to check knowledge level of both juniors and experienced professionals.