If you've ever been interested in web development, you've probably heard that Node.JS is often mentioned when discussing JavaScript and back-end development. This tool has received a lot of attention lately. If you are a business owner and would like to use Node.JS as a software development tool – great! This guide of preparing questions for interviews with Node JS developers is exactly what you need!
25 Most Asked Node.JS Interview Questions
In this guide, you will find some of the most popular interview questions and answers on Node.JS that you can ask your potential employees. We will cover basic questions regarding JavaScript or Node itself, as well as complex questions about internal development, and help you to prepare for the interview!
Introduction
Looking for a partner to develop a mobile app?
To be consistent, we will start the article with an overview of the basic questions and answers to Node.JS interviews that you can ask junior developers. These questions can be asked at the beginning of the interview, after the candidate introduced themselves and spoke a little about themselves.
First of all, this is a really good tactic to get to know the developer as a person. The way they answer questions, where they stop and start thinking, and other small details like this, can actually say a lot about the developer, especially about the amount of work they actually put into studying the subject.
Another reason why these basic Node.JS interview questions are so important is because you can check the basic level of Node.JS knowledge. After you evaluate the level of qualification of the candidate, you can decide what type of difficult questions you should give the developers later.
In general, it’s important that you don’t discard the main questions just because they can be “easy” or “not important”. The fact of the matter is that the questions in the interview about the entry-level Node.JS can actually be more important than the advanced ones – in the end, they determine the course of the rest of the interview.
Question 1: What is Node.JS?
Of all the basic questions about Node.JS, this is certainly the main one. Naturally, this is also often the first or one of the first questions that you can ask in an interview.
Even experienced Node JS specialists sometimes cannot answer this question smoothly. When a developer is completely immersed in the work on Node.JS, they do not always think about what it is. It’s often quite difficult to define this tool in one short sentence.
Node JS is a JavaScript-based tool designed to perform internal development and implementation processes.
We can say that this is the most affordable version.
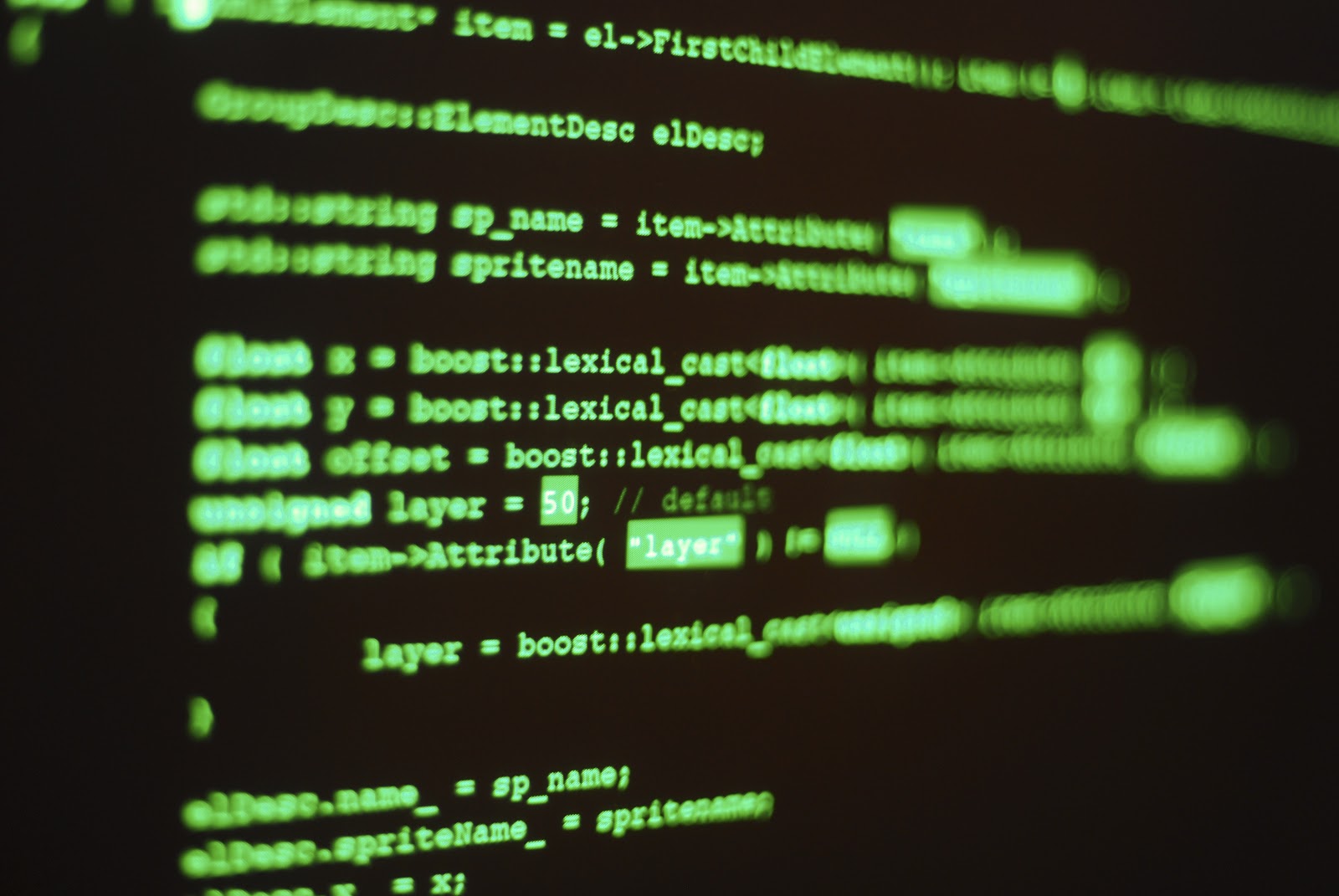
Question 2: Why do we need to use Node.JS?
One of the more subjective interview questions about Node JS. The candidate should express their opinion about the tool and its benefits. Since there are quite a few of them, most likely the candidate will describe those that interest them or bring them the greatest benefit.
Some examples of possible answers that you can get: it is fast, asynchronous, provides a single, generalized programming language and data type, etc.
Overall, Node JS is just one of the best tools on the market when it comes to server-side development based on JavaScript.
Question 3: What is the difference between front-end and back-end development?
This is one of those Node.JS questions that may catch the developer by surprise, as it is not directly related to Node JS. This question should be one of the easiest for developers. It all comes down to how they would formulate the answer.
Front-end developers are engaged in the client (user) part of the web page. They work, develop and support everything that the client sees – in other words, they are responsible for the visual (design) and functional (for example, buttons, banners, etc.) parts of the page. In contrast, backend developers focus on processes running in the background – something that customers don't see. They are also responsible for the functionality of the site, simply from a broader perspective.
Question 4: What are stubs?
Stubs are specific functions that mimic the behavior of certain modules. They are most often used in the test, because they can provide the answers necessary to solve problems that may arise in the modules.
Question 5: Describe callback hell
An interesting term in itself, callback hell occurs when a huge number of callbacks add up to one specific place, thus, it becomes impossible to read and – in general – work with it.
The candidate may also mention that callback hell can actually be resolved. This is done through a modular process. It works so that this process simply breaks down callbacks into separate functions that are independent of each other.
console.log("Pyramid of dooooooooom!")
step1(function (value)) {
step2(function (value)) {
step3(function (value)) {
step4(function (value)) {
step5(function (value)) {
step6(function (value)) {
step7(function (value)) {
//Do something with value 4
console.log(value7)
});
});
});
});
});
});
});
Question 6: What is an Event?
Events are one of the core features of Node JS. They symbolize some action taken or done on a web page. They are controlled by an event handler that writes the code necessary to execute these events.
Question 7: What is event-driven programming?
Previous questions raised about events and callbacks, so this should be a fairly simple question at this point.
Event-driven programming, as the name implies, is a form of programming that is associated with and based on events. Whenever an event occurs, callbacks are sent to the main server, which in turn retrieve the information needed for that particular event.
Question 8: What is the meaning of single-threading Node.JS?
This is one of the top node.js interview questions, as it requires the developer to know both the benefits of single-threading, and what it really is.
Single-threading allows Node JS to perform asynchronous processing. If you work with the default web download, single-threading provides a smoother and faster workflow – this is exactly what web developers are looking for!
Question 9: What is worker processes?
Worker processes are just those processes that are running in the background while you are doing something else. They can send emails, set variables and so on. They are extremely useful because they save a lot of time for web developers by completing these monotonous tasks.
Question 10: What is Express JS?
This is another interview question on Node JS that is not directly related to any Node feature. However, it is still important to know what Node JS Express is, as it is explicitly designed for Node JS.
Node JS Express is a lightweight framework designed to help Node deal with some of the most tedious web development tasks. This is a great help in developing web pages and mobile applications.
Question 11: What are some commonly used timing features on Node JS?
The most commonly used timing functions of Node.js are setTimeout() and setInterval(). The function setImmediate() isn`t so popular.
Question 12: How many types of API functions are there in Node JS?
The correct answer shouldn’t scare you at all, as there are only two types:
1) Asynchronous
2) Synchronous
Question 13: What is the purpose of module.exports?
This instruction serves to specify parts of the module that should be accessible from other files.
Intermediate Node JS Questions
Question 14: What is an event-loop Node JS?
Event loop is what allows to perform non-blocking operations. This is an endless loop in NODE.js, waiting for the tasks, executing them and sleeping till the moment it gets other tasks. It’s behind JavaScript’s asynchronous programming and it performs tasks on a single thread, but as it applies few data structures it looks like multi-threading.
Question 15: How can we use async await in Node JS?
We could use an async keyword to define a function that will return a promise or any value wrapped in a promise.
Keyword await we could use to wait for the promised result.
The combination of those keywords allows us to write more readable code than with the callback method.
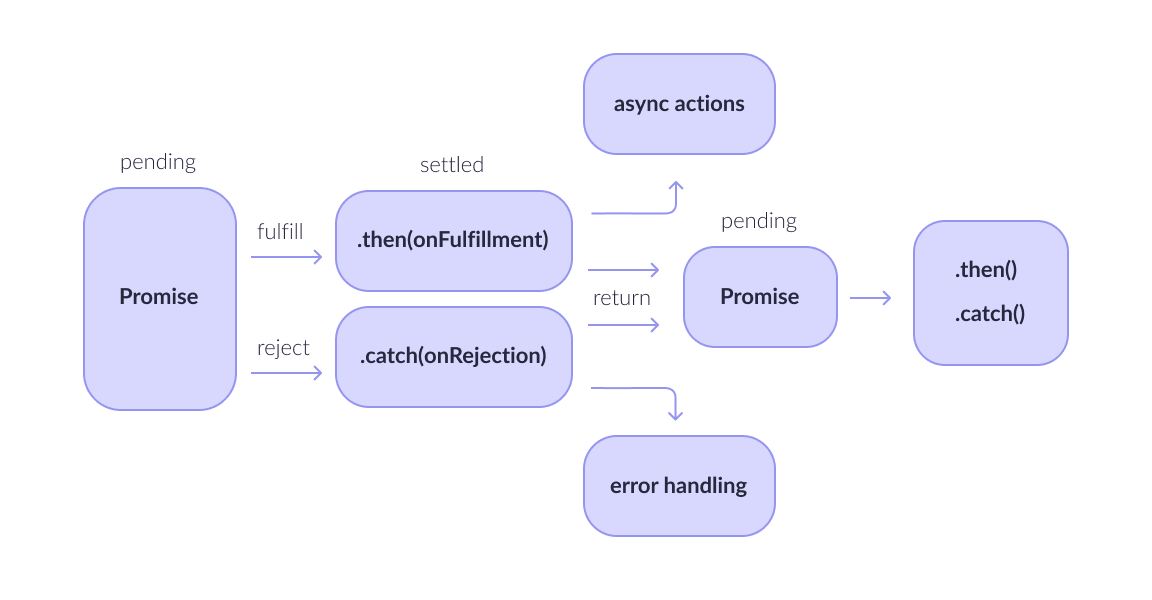
Question 16: What are node.js streams?
Streams allow to load data partially and don't store the whole set of data (file, network data, etc.).
They could be for read only operations (Readable), write (Writable), read and write (Duplex) and for update data (Transform).
Question 17: What are node.js buffers?
Node.js buffer is a class that serves to allocate fixed(not resizable) amount memory for some binary data.
Question 18: What is middleware?
Middleware is used to process requests. They are executed like chains of functions during the request life cycle(actually implements pattern chain of responsibility).
Advanced Node JS Questions
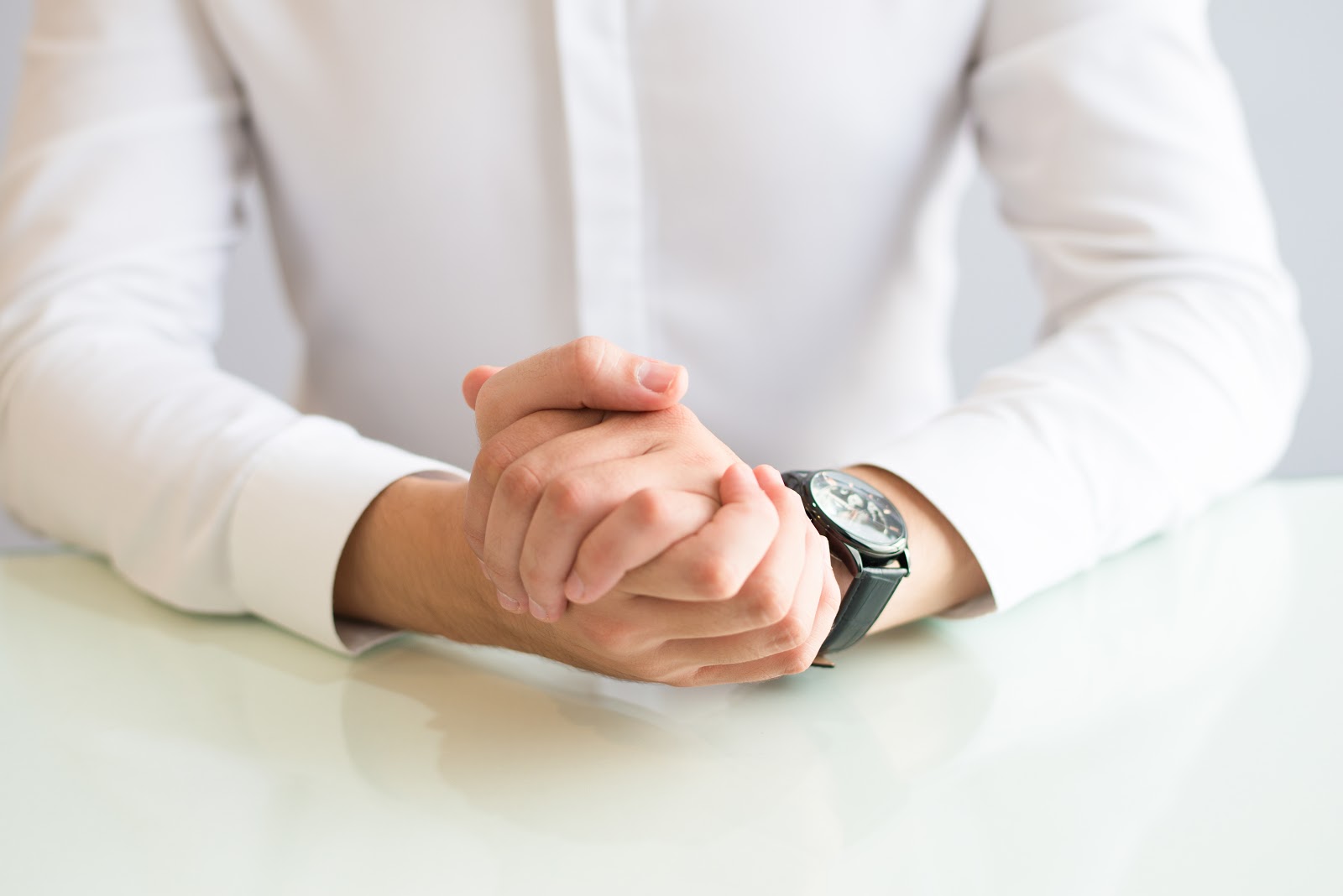
Now that we’ve covered some of the popular Node.JS beginner interview questions, let's move on to the advanced Node.JS interview questions and answers for experienced web developers.
Question 19: What is a demultiplexer?
Although this is simply a question from the “what is ...?” category, it does refer to this section of advanced interview questions for Node JS. This is not a term that a developer has often come across, especially if they are just starting out with Node JS.
The demultiplexer is a notification interface in the JS host. It is used to collect information about specific events and form quests, thereby providing the so-called Event Que.
Question 20: What is REPL and what does it do?
REPL stands for Read, Evaluate, Print, Loop. This shell is used to execute certain JavaScript statements.
Question 21: What is the difference between blocking and non-blocking functions?
When you start the block function, all other parts of the code are not applied until a specific, assigned I / O event is completed. In contrast, non-blocking functions allow developers to perform several tasks (maintain the health of several different codes) while simultaneously performing a series of I / O events.
Question 22: Does Node JS have sub-branches?
Some Node.JS interview questions can catch the developer off guard – this is just one of them.
If the initial answer was “no,” unfortunately, the candidate is mistaken. Although Node JS is a single-threaded service, it still has child threads – it just does not display them to the developer. So such tricky questions will also help you evaluate the candidate’s understanding!
Question 23: What is the name for implementing security in Node JS?
NPM phishing and regular expressions Denial of Service (DoS) is included by most Node JS security issues.
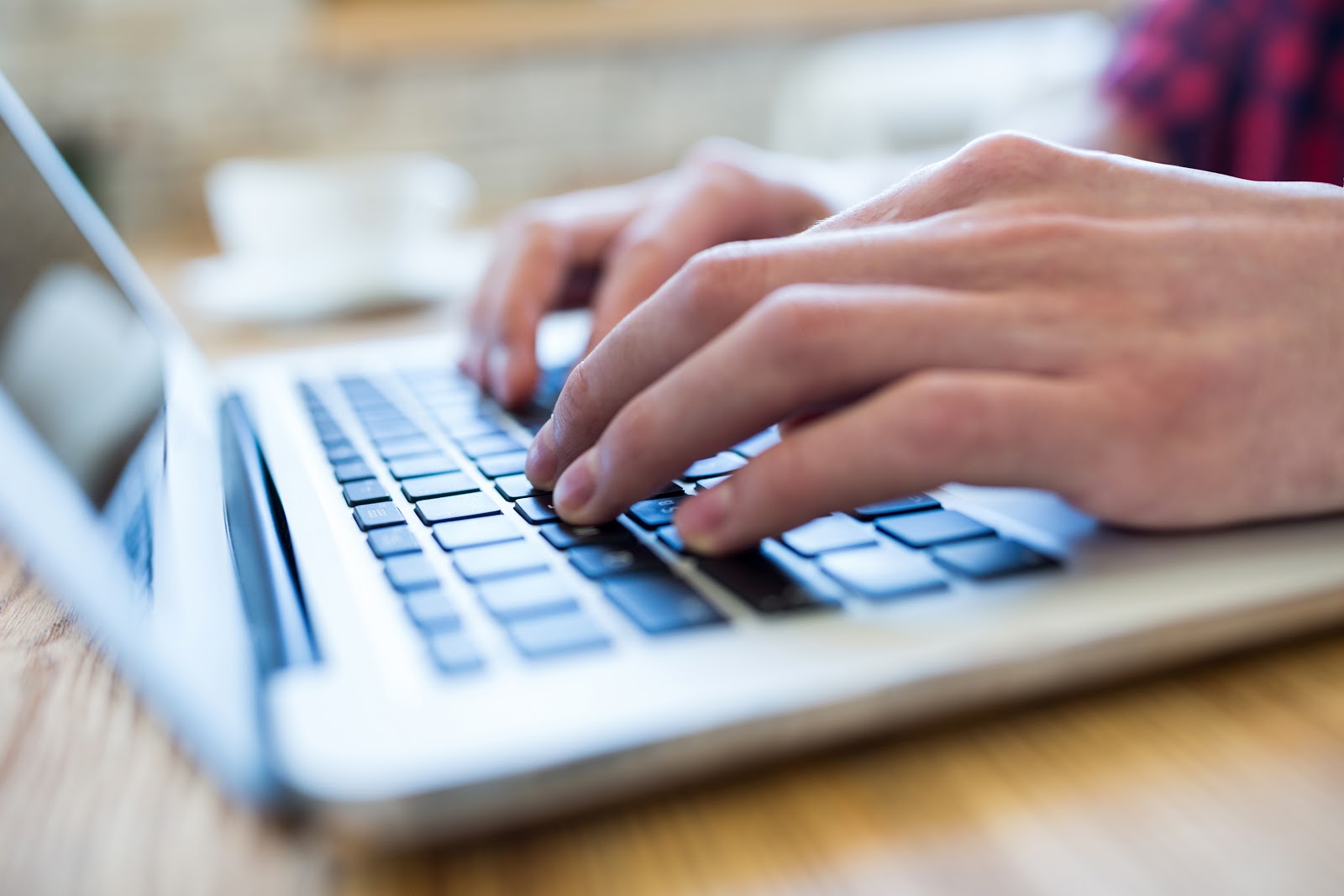
Question 24: How to measure the duration of async operations?
This is one of the most challenging Node JS interview questions.
With keyword await it is simply the difference between the time that was before and after execution (could use new Date for example, or for better precision hrtime). In the second case, we need to call then() function to find the difference between these two values (just define some time mark before operation and a new time mark inside the function).
Question 25: What is an Event Emitter in Node JS?
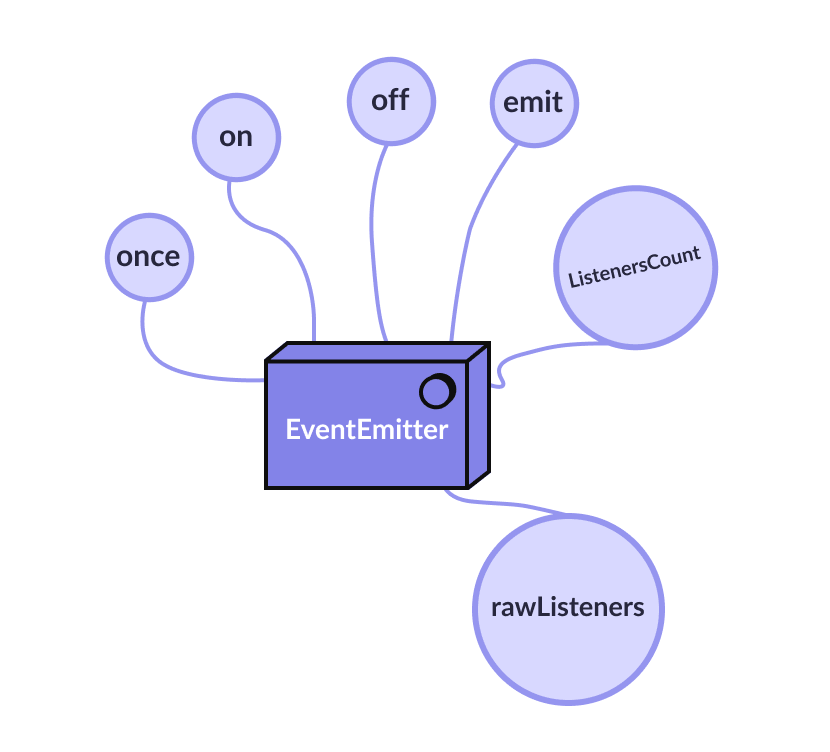
Event Emitter is a special module that smooths the path of communication between objects in NODE.js. It is at the core of asynchronous event-driven architecture of NODE. An event emitter is a pattern that listens to a named event, fires a node.js callback, then emits that event with a value.
Conclusion
Node.JS is truly one of the most useful web development tools of late. It is based on JavaScript and allows you to be flexible and easily adaptable to most web development scenarios.
If you want to hire a Node JS specialist, you will need to know what questions you can ask. If you study the questions and answers presented in this guide, you should get a pretty good idea of what you can expect from the candidate.
I hope you can scale your business and succeed in interviewing! Good luck!