We would like to share an approach that we have been using for many years in applications development, including web applications. Many developers of desktop, server and mobile applications are familiar with this approach. It is fundamental while building such types of applications. However, it is very poorly represented on the web. At the same time, there are definitely those who want to use this approach.
What is Clean Web Application Architecture and How to use It in Web Apps?
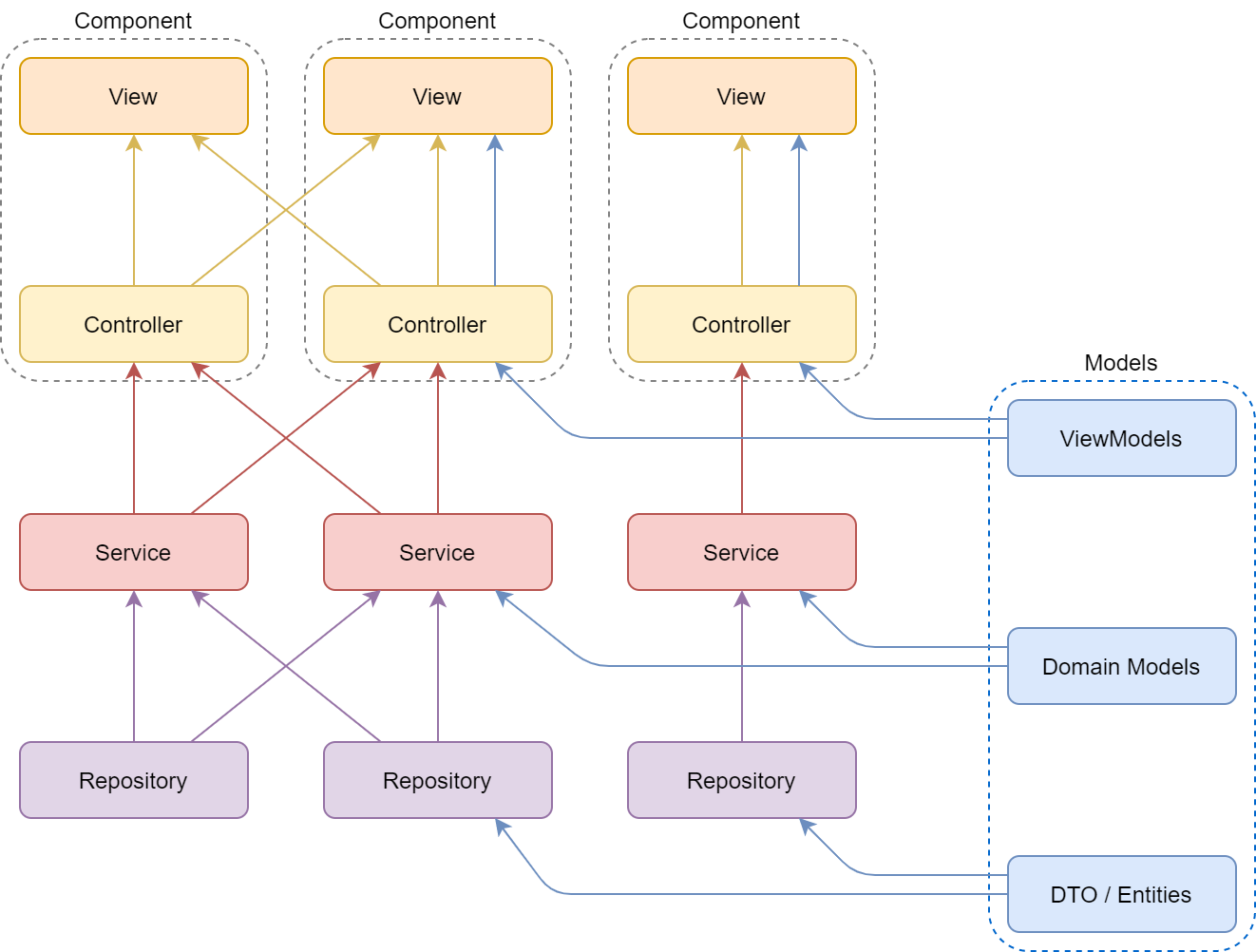
As a result of applying this approach, you will not be tied to a specific framework and will be able to easily switch the view library inside your application, for example, React, Preact, Vue, Mithril, without rewriting business logic, and in most cases even views. If you have an Angular 1 app, you can easily translate it to Angular 2+, React, Svelte, WebComponents, or even your view library. If you have an application in Angular 2+, but there are no specialists for it, then you can easily transfer the application to a more popular library without rewriting the business logic. And in the end, forget about the problem of migrating from framework to framework altogether. What kind of magic is this?
Levels of web application architecture
There are four general tiers of web applications. Below we will take a closer look at each of them.
Presentation Layer (PL)
PL displays the user interface and simplifies user interaction. The presentation layer has user interface components that render and display data to users. There are also user process components that define the interaction with the user. PL provides all the necessary information to the client side. The main purpose of the presentation layer is to receive input, process user requests, send it to the data service, and display the results.
Data Service Level (DSL)
The DSL transfers data processed by the Business Logic Layer to the Presentation Layer. This layer ensures data security by isolating business logic from the client side.
Business Logic Layer (BLL)
BLL is responsible for the proper exchange of data. This level defines the logic of business operations and rules. Site login is an example of the business logic layer.
Data Access Layer (DAL)
DAL offers simplified access to data stored in persistent stores such as binaries and XML files. The data access layer also controls CRUD operations - create, read, update, delete.
Types of web application architecture
Microservice architecture
Modern web applications are built on a microservice architecture, a style that structures an application as a collection of services. Each such service is as autonomous as possible, it is necessary for a specific task and is supported by a specific team. This architecture allows for a modular approach to building applications, taking into account the needs of the business. In other words, you can always change one or more services, such as a web server or a request server, if your needs change without compromising your business.
Monolith architecture
Another variation of the approach to building applications is a monolithic architecture, which offers the advantage of minimal consistency issues, but does not have the same flexibility in building as a microservice architecture. Monolithic architecture has a right to exist, but its advantage is also its disadvantage, and in the modern world it is practically not found in web applications, since it does not meet the Time To Market requirements - the business requirements for the release of new products to the market.
An application built on this architecture is easier to manage, since it does not require a large number of development and maintenance teams, but it takes a lot of time and effort to refine an already released version and create a new one. The same applies to issues related to the search and elimination of vulnerabilities. We can say that there are no applications without vulnerabilities, since errors are common to all people, because it is people who write the code of these applications and collect their disparate parts into one whole.
What is Clean Architecture?
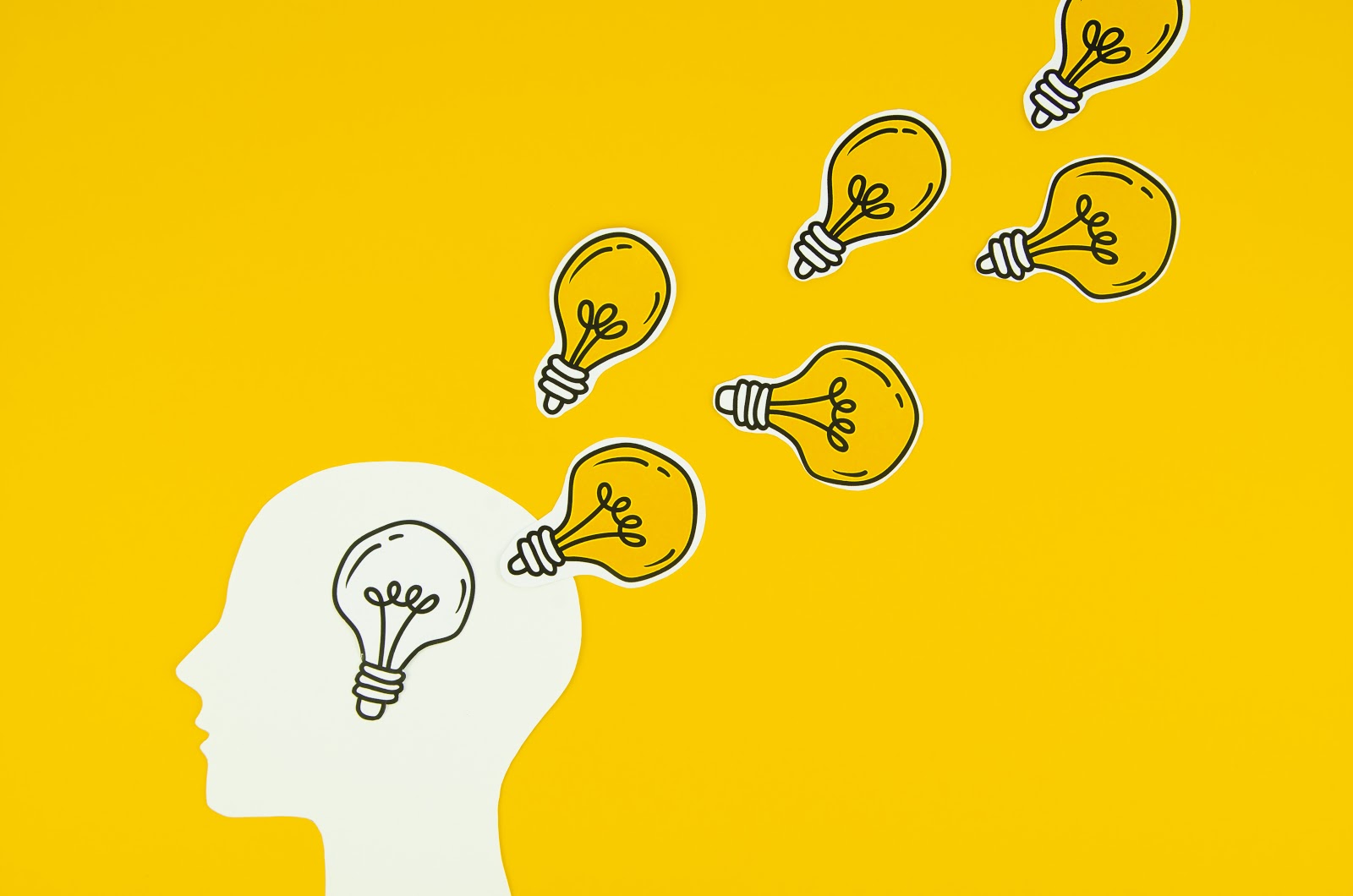
Framework independence.
The architecture does not depend on the existence of any library. This allows you to use the framework as a tool rather than squeezing your system into its limitations.
Testability.
Business rules can be tested without a user interface, database, web server, or any other external component.
Independent from UI.
The user interface can be easily changed without changing the rest of the system. For example, the web interface can be changed to a console one without changing the business rules.
Database independent.
You can swap out Oracle or SQL Server for MongoDB, BigTable, CouchDB, or whatever. Your business rules are not database related.
Independence from any external service.
In fact, your business rules simply don't know anything about the outside world.
For many years, the ideas described in this book have been the basis for building complex applications in a wide variety of areas.
This flexibility is achieved by dividing the application into Service, Repository, Model layers. We added the MVC approach to Clean Architecture and got the following layers:
- View - displays data to the client, actually renders the state of the logic to the client.
- Controller - responsible for interacting with the user via IO (input-output).
- Service - is responsible for business logic and its reuse between components.
- Repository - responsible for retrieving data from external sources such as database, api, local storage, etc.
- Models - is responsible for transferring data between layers and systems, as well as for the logic of processing this data.
Who is Clean Architecture for?
Web development has come a long way, from simple JQuery scripting to the development of large SPA applications. And now web applications have become so large that the amount of business logic has become comparable or even superior to server, desktop and mobile applications.
For developers who write complex and large applications, as well as transfer business logic from server to web applications to save on server costs, Clean Architecture can help organize code and scale the project to huge scales without problems.
At the same time, if your task is just layout and animation of landing pages, then there is simply no room for Clean Architecture. If your business logic is on the backend and your task is to receive data, display it to the client and process the click on the button, then you will not feel the flexibility of Clean Architecture. However, it can serve as an excellent springboard for explosive growth of the application.
Where is Clean Architecture already being applied?
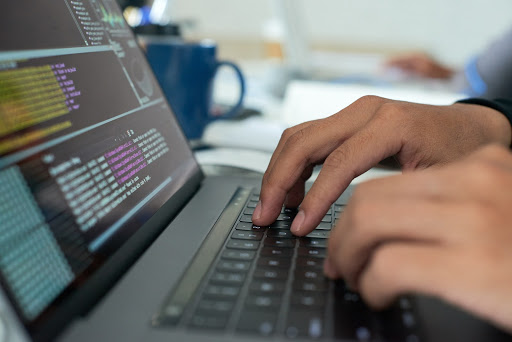
Clean Architecture is not tied to any particular framework, platform, or programming language. It has been used to write desktop applications for decades. Its reference implementation can be found in the Asp.Net Core, Java Spring and NestJS server-side application frameworks. It is also very popular when writing iOs and Android applications. But in web development, it appeared in an extremely unfortunate form in the Angular frameworks.
How to use Clean Architecture in web applications?
The only framework that tried to use Clean Architecture was Angular. But it turned out just awful, either in 1 or in 2+. And there are many reasons for this:
Angular is a monolithic framework.
And this is its main problem. Not only are there a lot of problem areas, but it also contradicts the ideology of Clean Architecture.
A terrible adaptation of the DI pattern.
It was simply moved as it is, without considering the peculiarities of web applications and ignoring the modular import system of modern Javascript.
Terrible view engine.
It is very primitive and falls far short of the simplicity of JSX. Data is not typed at the stage of writing the code, and at the stage of compilation. We learned to identify errors only in version 6, and before this version appeared we had managed to do that only in runtime.
Old bundler.
While the Rollup bundler allowed building ES2015 and making 2 bundles for old and new browsers for 4 years already, the angular builder only learned to do this in version 9.
And many more problems.
In general, modern technologies reach Angular with a delay of 5 years relative to React.
But what about other frameworks? React, Vue, Preact, Mithril and others are purely presentation libraries and do not provide any architecture ... and we already have the architecture ... the only thing left to do is to collect everything into a single whole!
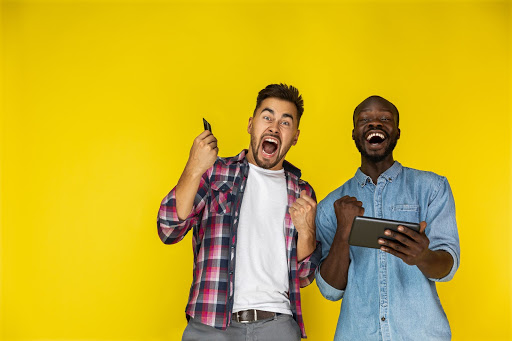
Clean Architecture Bonus 1: Expanding the functionality of the framework components.
As a bonus, we got the opportunity to supplement or change the behavior of components of the presentation library. For example, the React does not render the entire application on an error in the view. Using the Clean Architecture approach, the React will render not only the component in which the error occurred. In the same simple way, you can add monitoring, analytics and other features.
Clean Architecture Bonus 2: Data Validation
In addition to describing data and transferring data between layers, models have a large margin of capabilities. For example, if you connect the class-validator library, then simply by hanging decorators you can validate the data in these models, incl. with a little tweak, you can validate web forms.
Clean Architecture Bonus 3: Create Entities
In addition, if you need to work with a local database, you can connect the typeorm library and your models will turn into entities by which the database will be generated and operated.
If you like this article - share it with your friends and like-minded people?